I’ve got an Arduino UNO board, and a Hitachi HD44780 type LCD. I wanted to write a python program to communicate with the Arduino board. The board can be connected to the computer via USB, and it appears as a COM port. Therefore we can easily communicate with the Arduino serial interface with python.
My aim is to create a python program that takes the input from the keyboard and display on the LCD. The LCD is connected to the Arduino board as mentioned in the Arduino example:
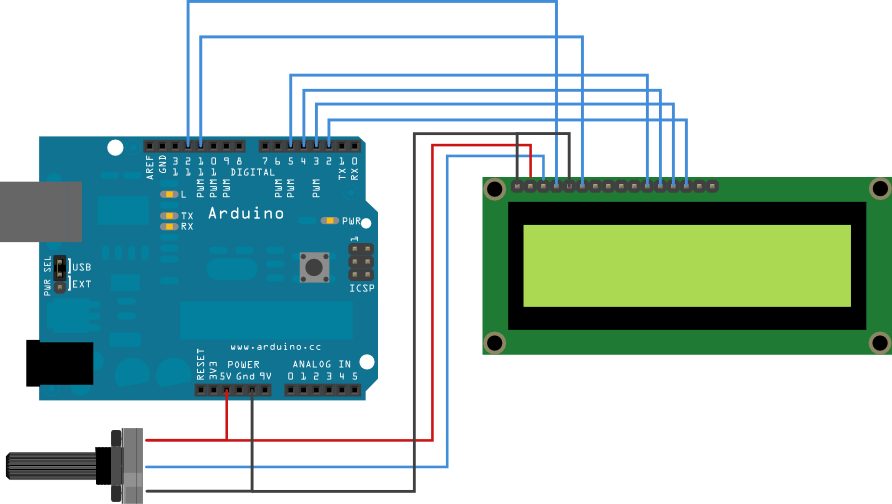
Circuit Diagram
The following is the code for the Arduino:
// include the library code: #include <LiquidCrystal.h> // initialize the library with the numbers of the interface pins LiquidCrystal lcd(12, 11, 5, 4, 3, 2); void setup() { Serial.begin(9600); lcd.begin(16, 2); lcd.print("start"); } void loop() { if (Serial.available()) { delay(100); //wait some time for the data to fully be read lcd.clear(); while (Serial.available() > 0) { char c = Serial.read(); lcd.write(c); } } }
To access the serial ports you need to set up the pySerial module for python. The Python code:
import serial import time s = serial.Serial(11, 9600) #port is 11 (for COM12), and baud rate is 9600 time.sleep(2) #wait for the Serial to initialize s.write('Ready...') while True: str = raw_input('Enter text: ') str = str.strip() if str == 'exit' : break s.write(str)
I’m on a windows machine, and by default the Arduino is connected to COM12. Note that we need to wait for a little while after initializing the serial connection.